One of the luxuries of using an open source programming language like Python is that you have a lot of options for running your code. Compare this with proprietary software:
- xlsx spreadsheets: open with Microsoft Excel
- m-files: run with MATLAB
- JSL script: run with JMP data analysis software
You are kind of limited to the interface provided by the parent company. For better or for worse, Python has the opposite problem. There are many ways to both write and run Python. Knowing which to use can be confusing at first, especially for folks who are not software developers by training. This post will show modern methods for writing and running Python. Let's start with a simple example.
Writing your fist Python code can be as simple as writing:
a = 1
b = 2
c = a + b
print('a + b is', c)
This is an unrealistically simple example. Useful python code that achieve a desired task will be longer and more complex. No matter how complex and long the code is, you still have to "run" it somehow.
There are several options and we have categorized them into 4 buckets:
- Terminal
- Terminal + Text Editor
- Software IDEs (short for Integrated Development Environments)
- Research and Development IDEs
Let's discuss them one at a time.
1. Terminal
There are kind of two options here: the Python interpreter and the IPython interpreter. The names are very similar, but trust me, a single "I" can make a big difference in improving your workflow. Let's see how the first option works.
a) The Python Interpreter
If you are using Windows 10, install Python through the Microsoft Store.
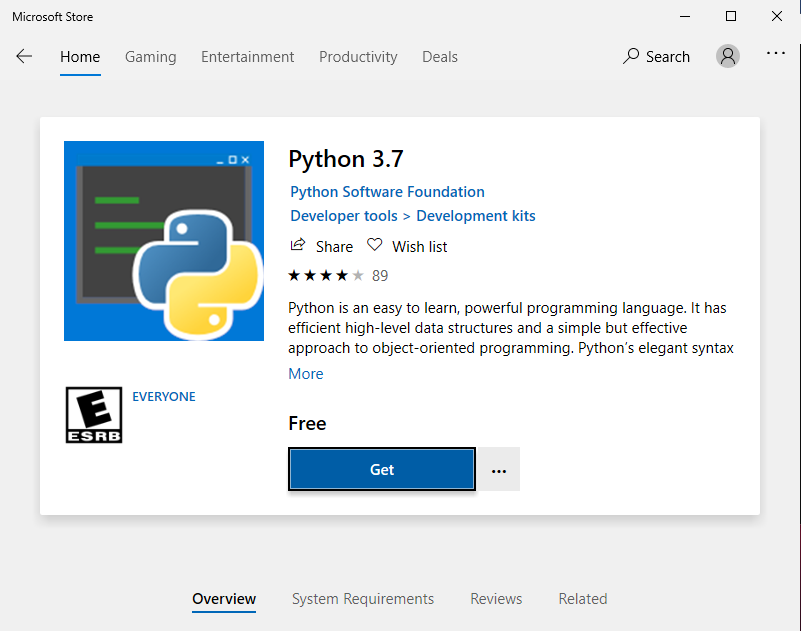
Then, open the command window by typing cmd
in the start menu. If you are using Linux (such as Ubuntu), you simply need to open a terminal, type python
, and press Enter. Python comes preinstalled on most Linux distributions.
Let's type in the above example code one line at a time.
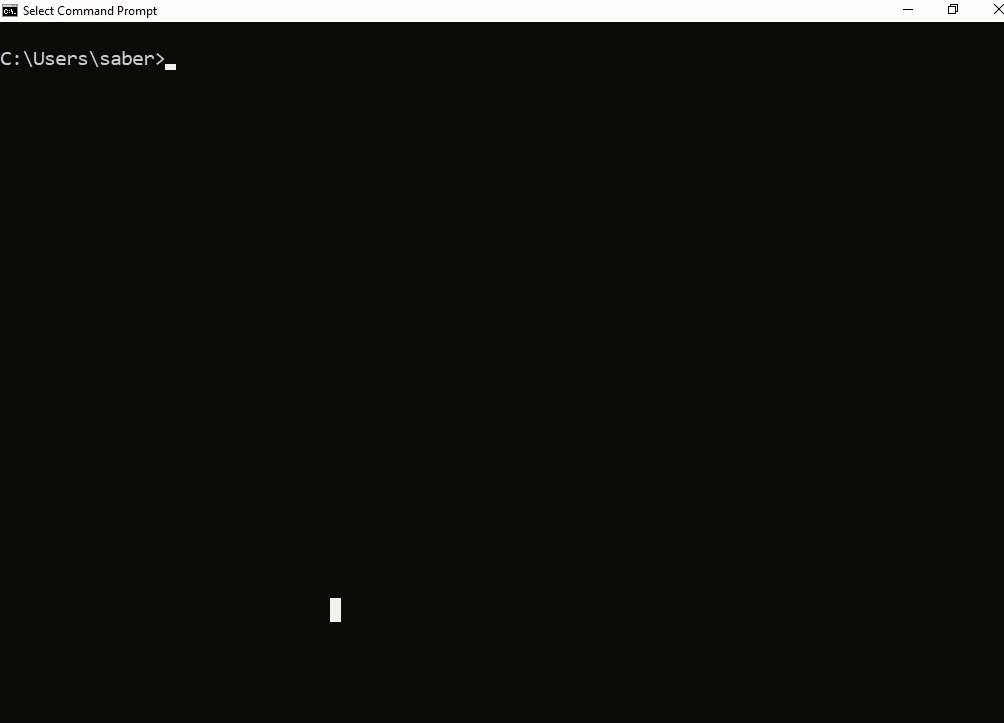
Congratulations! We ran our Python code interactively using the Python interpreter.
a) The IPython Interpreter
The IPython interpreter is like the Python interpreter, but with superpowers. It has a lot of additional features which are awesome for trying out ideas. Most of the advanced Python development environments, which we will discuss later, use IPython either directly or under the hood somewhere.
The easiest way to access IPython in Windows, iOS, or Linux, is to install the Anaconda Python Distribution. Once installed:
- Windows: open
Anaconda Prompt
from the start menu - Linux and iOS: open the terminal and type
conda activate
Then, type in ipython
and press Enter. This should bring up the IPython terminal. Now, we can enter the same example code. It will look pretty much the same for all operating systems. But to be fair, let's see this demonstration in Linux (Ubuntu).
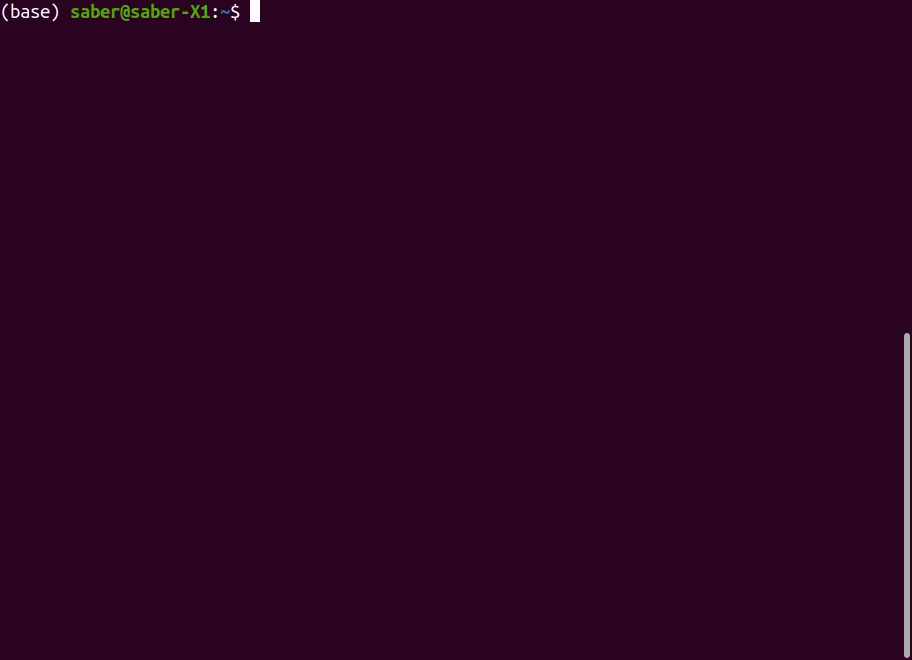
Just to show an immediate benefit with IPython, imagine the case where our variable names are not as simple as a
and b
:
q1_spending = 50
q2_spending = 60
total_spending = q1_spending + q2_spending
print('total spending is ', total_spending)
Using IPython, as you are typing in a variable, you can press Tab
and it will show suggestions or auto-complete the variable name. Here is an example:
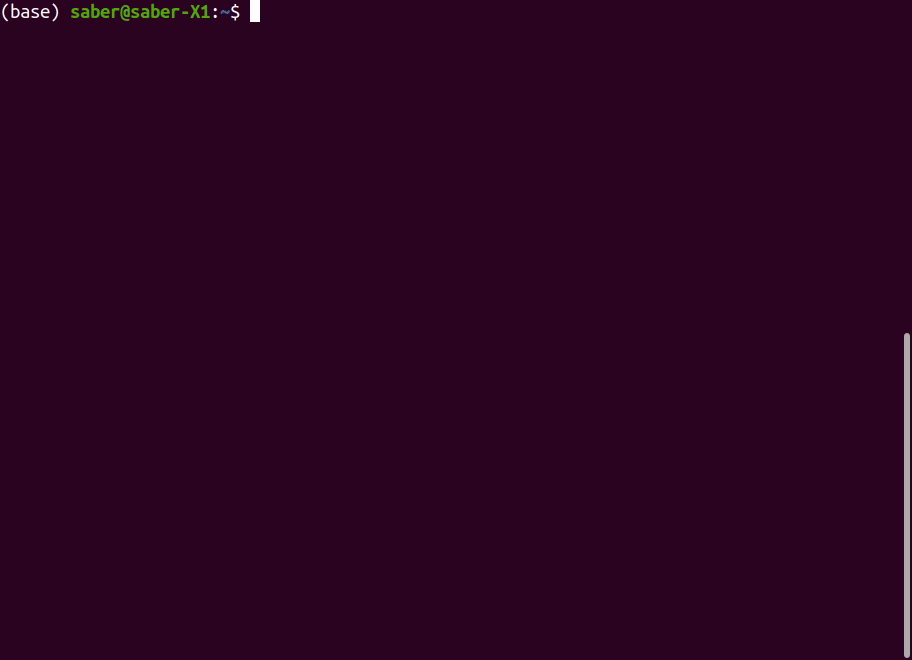
Using the terminal and the python interpreter to run the code is quite easy, but it has limitations. As your code gets longer, it become unreasonable to type it repeatedly. Or if a snippet of code is shared with you, you wouldn't want to copy and paste them one line at a time. Instead, you would collect these lines of code into a script. This brings us to the 2nd category of methods to write and run Python. In fact, the remaining methods are what you would be using for serious projects.
2. Terminal + Text Editor
Lines of Python code can be collected (or written) into plain text files, typically saved with the .py extension. Once written, the Python file is executed by pointing the python interpreter to it.
The main advantage with this approach is that you can use your favorite text editor such as Atom, Notepad++, Sublime Text, Visual Studio Code, or even Notepad if you are caught in a bind. Many of the fancy text editors have plugins that create a smoother experience for writing Python, like syntax highlighting or code completion.
Let's put our earlier example code into a file named my_code.py as follows:
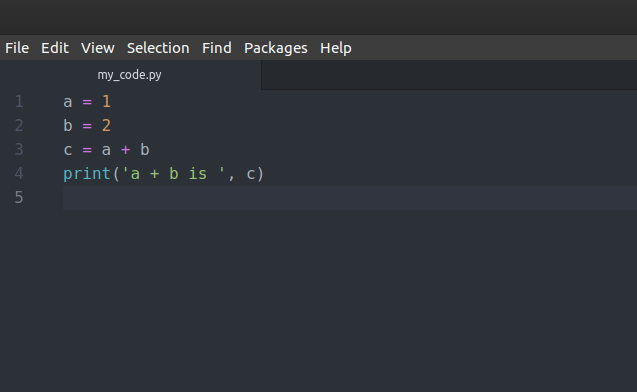
Then we can run this file in the terminal (or the Command Prompt in Windows) using the Python interpreter:
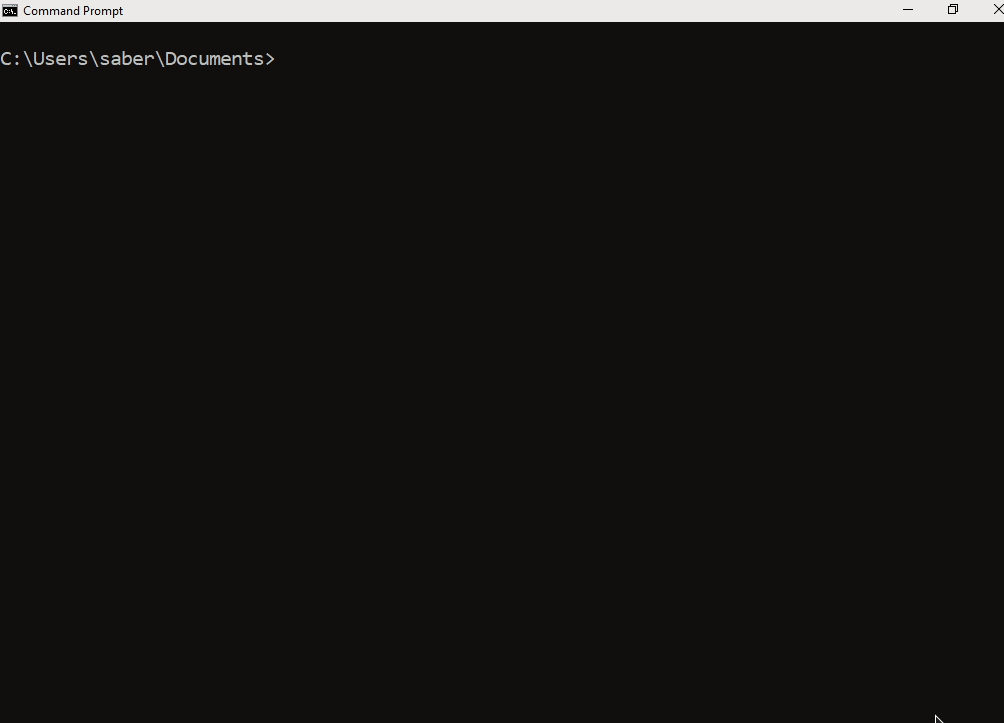
Now that we've separately introduced the Python interpreter and a text editor, it is time to combine them. The next category of environments for writing and running Python fuses these into an integrated development environment or IDEs.
3. Software IDEs
Software IDEs are where most software engineers spend their day. The same IDE will often support multiple languages (e.g. Python, Java, C++) and have a rich set of development tools like build scripts, debugging, profiling, version control integration, jump to definition, and code completion. If you have heard of Microsoft Visual Studio, it is a perfect example of a Software IDE. It can be set up to work with Python and more information is available here. Another popular software IDE for Python developers is PyCharm. Software IDEs can be very useful, or even absolutely necessary, for working with large and complex software projects with thousands of lines of code and dependencies.
There are cases where all the features of a complex software IDE are be unnecessary. Think of examples like laboratory data analysis, understanding a math problem, analyzing sales data, or developing a fraudulent transaction detection algorithm. The software might be quite simple but the complexity is in navigating the domain-specific problem. This brings us to the last category of environments which empower this class of users.
4. Research and Development IDEs
This bucket of IDEs enable interactive code execution, thus making it easier to try things and to gain insight into the problem at hand.
For instance, let's say that you have a manufacturing quality data set. You are trying to understand the relationship between process parameters (e.g. temperature, rail velocity) and the quality (pass or fail). These environments allow you to take a step-by-step approach:
- first load the data
- then do some plotting
- explore some correlations
- maybe go back and do more plotting
- and then if things look good, you can clean up your code for future reuse.
The popular options here are Spyder and the Jupyter Notebook. The latter option is particularly special in that it allows you to mix python code, documentation, equations, and plots all in a single notebook file.
In our Getting Started with Python for Data Analysis online workshop we show how to setup Jupyter for data analysis. Once the environment in setup, you can run code as cells, edit existing cells, add new cells, run all the cells, and so on.
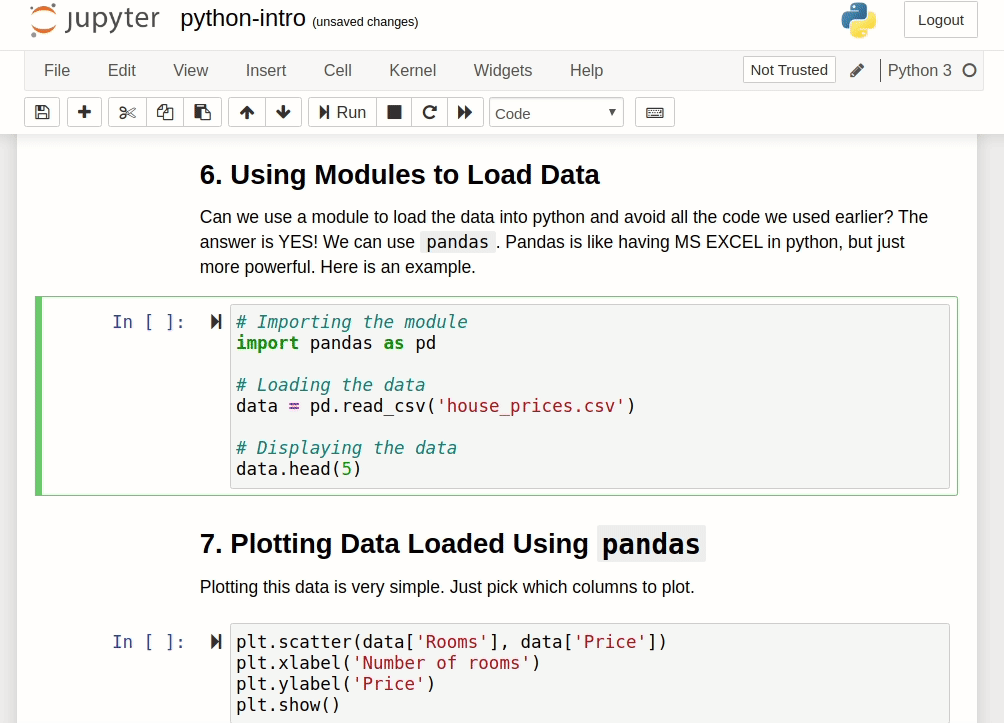
A number of advanced text editors have add-ons that run Python code within the editor itself, such as installing the Hydrogen Package for the Atom text editor. The PyCharm software IDE has a scientific mode designed to simplify this type of research and development (or science) workflow.
Conclusion
This covers it! Four categories of methods for writing and running python. Each category has a few options, so tens of ways to write and run your code. Hopefully one of the methods speaks to you and this post helps you get started in using it.